C++ Programming
Course Materials
Introduction to the Course
I, Philipp Schubert (/Philipp Dominik Schubert), have designed and created the "C++ Programming" course from scratch in WS 2016/2017. The course is intended to teach the C++ programming language, in particular, but it will also help to deepen your understanding of computer programming and the machine, in general.
The C++ (and also the C) language is not for everybody and requires a special kind of toughness. And the C++ programming language is huge. I try my best to give a useful and complete overview on the language. However, you need to learn and study the details on your own. The exercise sheets and final project will certainly help to deepen the contents and promote you to become a better programmer. Do not expect to become a pro only by attending this course--the rabbit hole is much deeper than you think. But with this course I aim at setting you up to become a real software engineering professional.
Unfortunately, I currently do not have the time to teach this course in person. Please use the course materials for self-study.
I wish you all the best and have fun with this course.
If you do have any questions, wish to criticize the course or provide any other kind of feedback, feel free to send me an email.
Course material
The lecture recordings are available on YouTube at Secure Software Engineering.
Materials
The gtest framework example shown in the exercises: gtest_example.zip
For details please refer to: https://github.com/google/googletest
Compiler builtins for over-/ underflow detection:
- Clang: http://clang.llvm.org/docs/LanguageExtensions.html#checked-arithmetic-builtins
- GCC: https://gcc.gnu.org/onlinedocs/gcc/Integer-Overflow-Builtins.html
VirtualBox machine image containing an Ubuntu OS with different C++ compilers (gcc, clang) and development environments (VS Code, etc.) pre-installed:
- cppp21.ova
- password: 'cppp'
To run the virtual machine you need to install VirtualBox. Please download the Virtual Box plattform package for your corresponding operating system (and install it). You probably wish to install the VirtualBox Extentsion Pack as well. Once VirtualBox is installed, you can run it and import the virtual machine (.ova file): In the 'File' menu of VirtualBox please choose 'Import Appliance' and follow the instructions. After having successfully imported the machine you should be able to start it and a new window with the Ubuntu OS booting should pop up.
Final Project
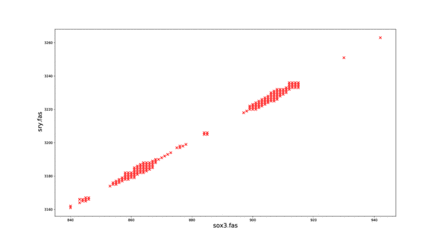
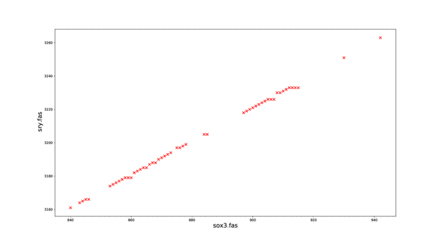
The project description can be found here: cppp_final_project.pdf
The compressed sequence files and a small python script for plotting the results can be found here:
You can use the file homo_sapiens_chr1_GRCH38.p7.fas to test the performance of your sequence-reading function. Use the sequences in sox3.fas and sry.fas for the actual comparison. If you are able to get a python interpreter installed on your system, you can plot the results of your program with help of the plotSMSresults.py script. Otherwise you can use any spreadsheet software such as Libre Office, Google Sheets or MS Excel to create scatter plots of your results.
If you have any problems solving the project send me an email and I will help you.
Plotting your results using the provided Python script should produce plots similar to the above (above without postprocessing, below with postprocessing).
Abstract
C++ is a general purpose programming language and one of the most popular languages for both, systems and application programming. The language allows for writing programs close to the metal and gives the programmer full control over the system's resources. However, C++ also includes decent mechanisms for abstractions as well. Another observation is that programs written in C++ often result in highly efficient machine code as the the language maps very well to the von Neumann architecture. These are just a few characteristics of the language which make it to be in great demand.
The more recent standards such as C++11, C++14, C++17, and C++20 (often called modern C++) provide new powerful mechanisms and concepts that make programming in this language a lot easier than a few years ago.
During this course you will learn the basics (and probably a lot more) about modern C++.
Prerequisites
The C++ programming language is taught from scratch, so students without any programming background are welcome!
Syllabus
This course teaches the following concepts:
Background and basic introduction:
- history of C and C++
- programming environments
- basic terms / concepts
Basic C++ programming:
- primitive variable types
- expressions / statements
- functions
- memory management / pointers / arrays
- structures / unions / enumerations
- strings / vectors
- classes
- smart pointers / move semantics
The C++ standard template library (STL):
- IO library
- containers
- generic algorithms
Advanced techniques:
- operator overloading
- template programming
- object-oriented programming
- embarrassing parallel: OpenMP
Useful libraries for further projects:
- commonly used C++ libraries
Learning outcomes
- understand modern C++
- be confident to take advanced courses that require C++ programming
- the ability to easily realize elegant programming tasks / projects
- promoted from a C programmer to a state-of-art C++ programmer
- the ability to develop a real object-oriented program
- gain additional understanding of how a computer works
- better understanding of (computational) problems
- know where to find the desired information to realize a challenging task on your own
Useful literature
[1] A Tour of C++, Stroustrup 2013 (overview)
[2] The C++ Programming Language (4th Edition), Stroustrup 2013 (details)
[3] C++ reference: http://en.cppreference.com (reference)
[4] C++ Tutorial: http://www.cplusplus.com/doc/tutorial/ (a nice tutorial)
[5] CppCon experts sharing their knowledge: https://www.youtube.com/user/cppcon (really advanced concepts)